Contribute to Hibernate Validator
Contribute to Hibernate Validator
You want to implement a new validation feature or you found a bug and want to provide a fix? Awesome! The following gets you started.
Requirements
-
JDK 7 (The HV binaries target Java 6 but JDK 7 is required for development as some test dependencies are based on Java 7)
-
Maven (>= 3.0.3)
Getting the source
The Hibernate Validator code is available on GitHub. To get a read only version of the source you can use:
git clone git://github.com/hibernate/hibernate-validator.git validator
For people with write access:
git clone git@github.com:hibernate/hibernate-validator.git validator
To contribute back to Hibernate Validator you should create a fork of the project into your GitHub account and submit pull requests with your changes. For more information on Git, check out this blog entry.
For those interested, the HV legacy code base (3.x) can be accessed under the pre-validator3-removal tag.
The source code of the Bean Validation API (of which Hibernate Validator >= 4.x is the reference implementation) can be retrieved via
git clone https://github.com/beanvalidation/beanvalidation-api
Finally there is the Bean Validation TCK (test compatibility kit) which can be checked out via
git clone https://github.com/beanvalidation/beanvalidation-api
Hibernate Validator modules
The Hibernate Validator code base comes in form of a multi-module Maven project, comprising the following modules:
-
annotation-processor: the HV annotation processor for checking correctness of constraints at compile time
-
cdi: the CDI portable extension
-
distribution: builds the released HV distribution package
-
documentation: builds the HV reference guide
-
engine: the core validation engine
-
integration: in-container integration tests
-
performance: a performance test suite based on JMeter
-
tck-runner: executes the Bean Validation TCK against HV
Building from Source
Compiling and testing
Prior to setting the project up in any IDE it is recommended to trigger a command line build to verify that everything builds. Just run:
mvn clean install -s settings-example.xml
This will download all dependencies and compile and test each module. settings-example.xml is just an example Maven settings file containing the required JBoss repository settings. You can also add the required settings to your own settings file under ~/.m2/settings.xml file. Refer to this article to see how to tell Maven to use the JBoss Maven repository.
Build options
There are several options/properties available to control the build. The following options might be useul to speed up the build time. Per default everything gets built!
Skipping parts of the build
The build of the distribution bundle (distribution) can be skipped via the disableDistributionBuild property:
mvn install -DdisableDistributionBuild=true
The build of the documentation module can be skipped via the disableDocumentationBuild property:
mvn install -DdisableDocumentationBuild=true
Deploying sources and javadocs jars as part of local install or snapshot deploy
In case you want the sources and javadocs jar installed as part of the build (either on a local install or a SNAPSHOT deploy) specify -DperformRelease=true
.
mvn -DperformRelease=true clean [install|deploy]
See also this SO post.
Staging Maven Release locally
Sometimes it is convinient to go through the Maven release steps without actually deploying anything. This makes sure the replease and deploy plugins work as expected and it gives you the chance to inspect the artifacts. One way of doing this is:
mvn release:prepare -DpushChanges=false
mvn release:stage -DpushChanges=false -DlocalCheckout=true -DstagingRepository=/path/to/a/local/directory
After you inspected all the artifacts you need to revert your local repository changes back to their original state:
git reset --hard HEAD~2
git tag -d <your-release-tag>
Writing and building the documentation
The documentation is written using Docbook and can be found in the module documentation of your checkout.
When writing documentation you should follow the JBoss Documentation Guide. This guide gives valuable recommendations about styling as well as general writing tips.
If you want to contribute to the documentation translation only you can get a Zanata account and just contribute your translation via Zanata. Have a look at Hibernate Search Documentation Translations. Everything mentioned there applies to Validator as well.
You can build the documentation by executing:
mvn package -pl documentation
To build the documentation including translations, po2xml must be installed (more information can be found here). You can skip the translation build by running:
mvn package -pl documentation -Djdocbook.ignoreTranslations=true
During developement it is often enough to build a single documentation output format, for instance html_single. You can just build this format by using the property jdocbook.format, skipping formats not specified:
mvn -Djdocbook.format=html_single install
IDE Setup
Eclipse
For Eclipse several plugins exist to integrate Maven projects. Probably the two most popular ones are m2eclipse and Eclipse IAM.
IDEA
IntelliJ IDEA come with built-in support for multi module Maven projects. Just import your project as Maven project and let IDEA scan recursively for modules. An IDEA code style template to be used for the Hibernate Validator code base can be downloaded here.
Running TCK tests
Running the Bean Validation TCK tests in the IDE can be a little tedious. One way is the following (should work in the same way in Eclipse as well as IDEA):
-
Create a new TestNG test configuration
-
Select the Suite option
-
Select tck-runner/target/dependency/beanvalidation-tck-tests-suite.xml as suite file. The Maven build extracts the suite file from the JSR TCK jar and places it into this directory
-
Specify the following VM options (you need to set the same properties as set by the Maven build, see pom.xml) :
-Dvalidation.provider=org.hibernate.validator.HibernateValidator
-DexcludeIntegrationTests=true
-
Select the hibernate-validator-tck-runner module as the project (Eclipse) or module to obtain the classpath from (IDEA).
All the steps are summarized in the screenshot below (using IDEA):
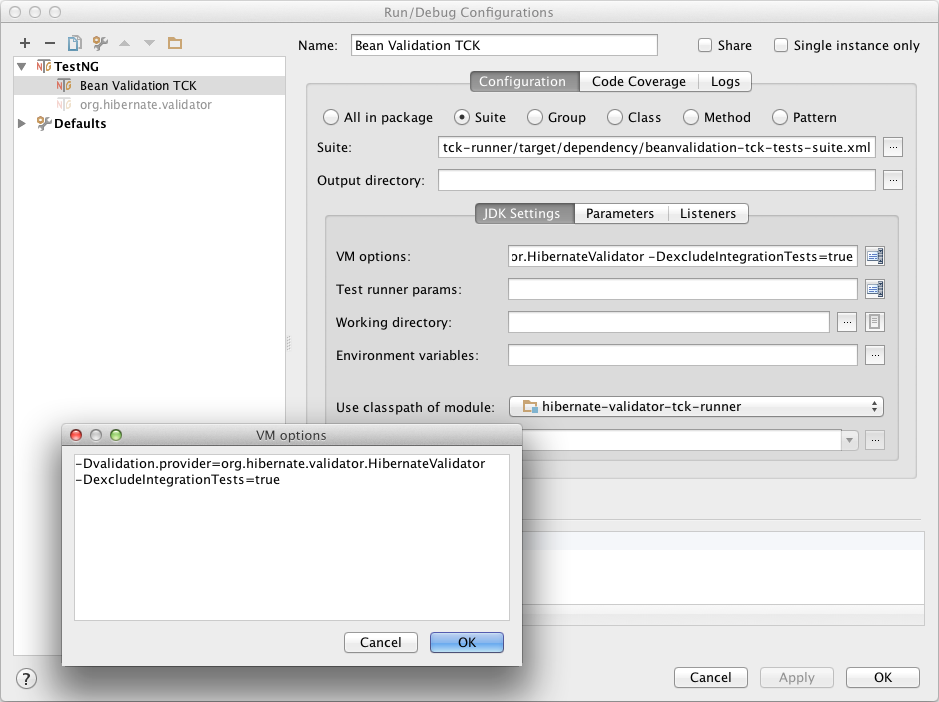
If you run this test configuration all TCK tests are getting executed. You can just edit the suite file to change which tests you want to run, e.g.:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd" >
<suite name="JSR-349-TCK" verbose="1">
<test name="JSR-349-TCK">
...
<classes>
<class name="org.hibernate.beanvalidation.tck.tests.validation.ValidateTest"/>
</classes>
</test>
</suite>
More information about how to confgure the TestNG suite file can be found here.
Coding Guidelines
General
Refer to the Hibernate design philosophy when working on new HV features. Hibernate Validator uses Java 6, so no Java 7 language features may be used.
Make sure to add the following license header to all newly created source files:
/*
* JBoss, Home of Professional Open Source
* Copyright 2013, Red Hat, Inc. and/or its affiliates, and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
JavaDoc
The following conventions should be followed when working on the Hibernate Validator code base:
-
Use
{@code}
instead of<code>
, because it is more readable and{@code}
also escapes meta characters -
@param
,@return
and@throw
don’t end with a .; the first word starts with a lower-case letter -
If referring to other classes and methods of the library, use
{@link}
-
{@link}
might be use for external classes,{@code}
is accepted, too -
Use
<ul/>
for enumerations (not -) -
Use the code style template mentioned above to format the code
Providing a patch
Patches including a test and fix for an issue are always welcome, preferably as GitHub pull request. We are following the Fork + Pull Model as described here. In oder to be able to integrate your patch you have to accept the JBoss Contributor License Agreement!